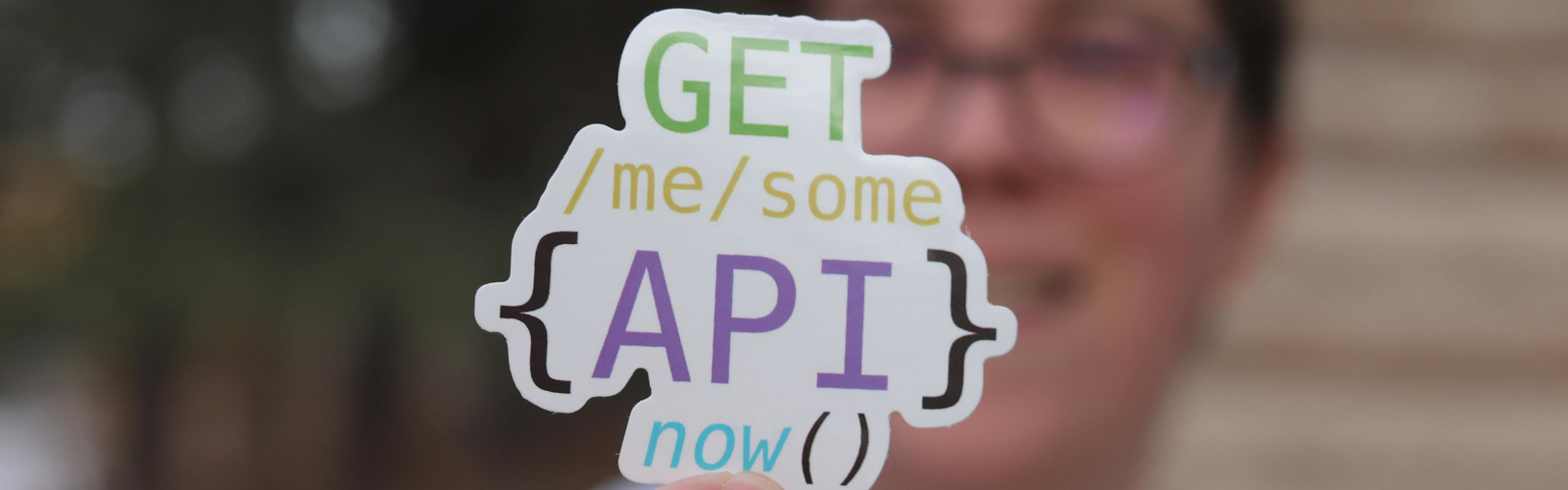
FastAPI vs Django

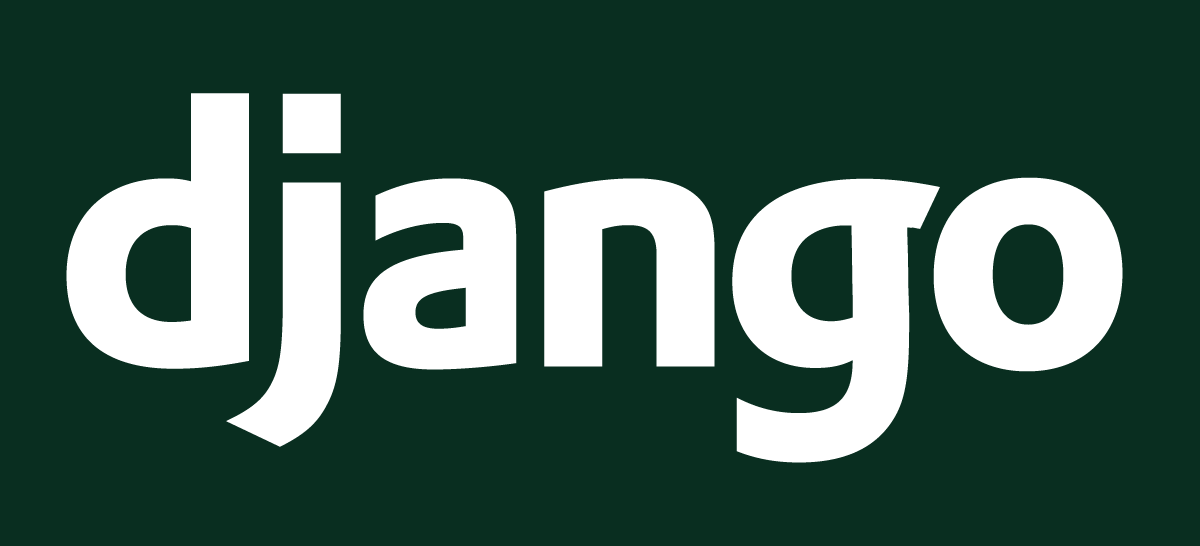
Lately we've been seeing increased attention towards FastAPI. Some people feel like it's a fad, yet there is some high-profile users. I want to do a comparison of FastAPI against Django. It's worth noting however, that FastAPI offers a different focus than than Django, as Django is more of a general purpose framework. However, there is enough overlap to make this worthwhile.
Keypoints
- Data Modeling is a bit different between the two
- Both provide Middleware
- Documentation is decent, but I like Django's better
Data Modeling
In my view, data modeling is one of the most important aspects of a web framework. A developer should be able to clearly and easily define a group of data, and then ship that out to the end user.
Django has its own Object Relational Mapper (ORM) that enables a developer to easily define database models/tables. The Django ORM also takes care of migrations, which makes things really nice when you need to update, change, or delete a database model/table. Also, Django ORM models are easy to learn and easy to implement. For example, here is a Django model that represents a database table to store different types of oranges:
from django.db import models
class Orange(models.Model):
species = models.CharField(max_length=32, db_index=True)
origin = models.CharField(max_length=32, db_index=True)
This is a basic unit of data modeling within Django.
However, in the FastAPI world, you are recommended to use SQLAlchemy and Pydantic.
To implement the same database model from Django in SQLAlchemy, the code would look like this (after creating the 'database.py' file for connectivity):
from sqlalchemy import Column, Integer, String
from .database import Base
class Orange(Base):
__tablename__ = "oranges"
id = Column(Integer, primary_key=True)
species = Column(String, unique=True, index=True)
origin = Column(String, unique=True, index=True)
Then you must create the Schema for Pydantic:
from pydantic import BaseModel
class OrangeBase(BaseModel):
species: str
origin: str
class OrangeCreate(OrangeBase):
pass
class Orange(OrangeBase):
id: int
class Config:
orm_mode = True
Creating API Endpoints
The default behaviour in Django is more geared towards the Model View Controller (MVC) style of programming. However, if you add the Python package called Django REST Framework, you can turn Django into a REST API powerhouse. The Django REST Framework has been around for years, and is pretty well documented.
For FastAPI, the default behaviour is to be an API. So you can more quickly create endpoints in FastAPI with less boilerplate code. Here is an example of a short FastAPI program:
from fastapi import FastAPI
from faker import Faker
app = FastAPI()
@app.get("/")
def root():
return {"name": Faker().name()}
Then if I visit this endpoint in my browser, I get this simple response:
{"name":"Clayton Pennington MD"}
However, using Django REST Framework, you typically would need to first create the function to process the request (which can also be a minimal amount of code), but then you must connect the function to a URL router. So there is an additional step there.
Middleware
Both Django and FastAPI provide middleware, and the process of creating custom middleware appears somewhat similar.
The default middleware that Django comes with is:
- SecurityMiddleware
- SessionMiddleware
- CommonMiddleware
- CsrfViewMiddleware
- AuthenticationMiddleware
- MessageMiddleware
- XFrameOptionsMiddleware
These pieces provide some default security, form security, user authentication, and a way to pass messages to views.
On the FastAPI side, we have middleware available, but maybe not all active by default:
- CORSMiddleware
- HTTPSRedirectMiddleware
- TrustedHostMiddleware
- GZipMiddleware
This is a good place to start though, as you can get some security gains with some of those.
Authentication
Authentication in Django is easy. There is literally 3 steps:
- Create the Django Process
- Run the migrations (you can use the default SQLite)
- Create a user
That's it. You have authentication right out of the box. You may need to add additional fields for the user profile, or tweaks to use an email instead of a username, but that's it.
If you go with Django REST Framework, then you can use Token authentication for API authentication. So authentication is a little more involved if doing just an API.
As for FastAPI, well, their documentation talks about implementing OAuth versions 1 and 2, JWT, and HTTP Basic auth. Plus, you need to consider linking the users to some sort of datastore. So there is some work required in order to get the basics of authentication working.
Documentation
I am seeing that both Django and FastAPI provide pretty good documentation.
Having used Django for a few years, I have never been truly disappointed in the documentation. It seems to provide enough details to get a decent grasp on whatever task I have needed. Also, for building API's with Django REST Framework, their documentation covers lots of topics as well.
When it comes to FastAPI, there is two great things: 1) decent documentation, and 2) automatic self documentation of the API.
When you build with FastAPI, the interface provides 2 documentation tools: Swagger & Redoc. This is incredibly helpful when you are building an API, because often the expectation is that a different developer will be building a mobile app or web frontend to connect to it. So having that documentation helps that developer implement a proper solution. So that is a big win.
However, you can implement Swagger or Redoc also in Django. It's just not a "default." Despite that, the documentation for Django has worked better for me personally. You might have a different experience though.
Conclusion
Django's claims to be: Ridiculously fast, Reassuringly secure, and Exceedingly scalable. FastAPI claims to be "high performance, easy to learn, fast to code, ready for production." I think both are true, but with their caveats.
Django can be used to build either a full website (with its own HTML), or an API. Django includes "batteries," such as a great ORM and additional middleware. Django feels very flexible, and includes tooling to build different types of services (website, API, etc).
FastAPI can be used to build an API. The data modeling is a little trickier with SQLAlchemy, as there is more manual steps. But it does come with important middleware. FastAPI feels more streamlined for a specific task, which is, building APIs.
And finally, although FastAPI does have automatic docs, it does not have an administrative panel like the Django admin. That alone, depending on the project, may just be a deal breaker. Giving the user access into their data is very helpful, and if there is no way to do that, then you need to build a way to do that, which could be a sizable chunk of labor.