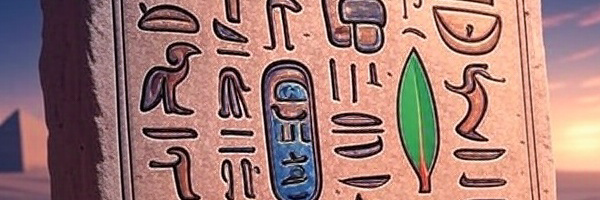
What Sort of Data Can MongoDB Hold?
Many times while I am programming in Python, I reflect back to the days of trying my hand at C and similar languages, and considering the strict data typing. In C you had to specify which type of data you were going to be using for a variable. Whether it was going to be a short or long integer, or a character, or multiple characters.
Well, Python does not have this limitation of specifying the data types. But, when you want Python to communicate with other components, you must start considering data types again. So a django web app with a PostgreSQL database specifies what sort of data each model is holding.
But then you have this other important concept: JSON. JSON is the modern standard of representing and transferring data. JSON doesn't have the same rules as a relational database system. These are the only types for JSON (from w3 schools):
- string
- number
- object (JSON object)
- array
- boolean
- null
So, what does this look like using the pymongo
module in Python?
Introduction to MongoDB Data Types
Of note, "MongoDB stores data using BSON, which supports additional data types that aren't available in JSON" (mongodb.com). MongoDB is a NoSQL database that allows for flexible and dynamic schema design. It supports a wide range of data types, including:
- Integers
- Boolean
- Double
- String
- Arrays
- Object
- Symbol
- Null
- Date
- Timestamp
- Binary data
- Object ID
- Regular Expression
- Code
Testing Data Types with Python
To test the various data types in MongoDB, we'll use the pymongo
library, which provides a Python interface to MongoDB. We'll create a simple Python script that inserts different data types into a MongoDB collection and then retrieves them to verify their correctness.
from pymongo import MongoClient
import datetime
# Connect to the MongoDB server
client = MongoClient('mongodb://localhost:27017/')
# Select the database and collection
db = client['test_db']
collection = db['data_types']
# Insert integers
collection.insert_one({'name': 'integer', 'value': 123})
# Insert float
collection.insert_one({'name': 'float', 'value': 3.14})
# Insert string
collection.insert_one({'name': 'string', 'value': 'Hello, World!'})
# Insert boolean
collection.insert_one({'name': 'boolean', 'value': True})
# Insert date
collection.insert_one({'name': 'date', 'value': datetime.datetime.now()})
# Insert timestamp
collection.insert_one({'name': 'timestamp', 'value': datetime.datetime.now().timestamp()})
# Insert array
collection.insert_one({'name': 'array', 'value': [1, 2, 3, 4, 5]})
# Insert object
collection.insert_one({'name': 'object', 'value': {'a': 1, 'b': 2}})
# Insert null and undefined value
collection.insert_one({'name': 'null', 'value': None})
collection.insert_one({'name': 'undefined', 'value': None})
# Retrieve and print the inserted data
for doc in collection.find():
print(f"Name: {doc['name']}, Value: {doc['value']}")
Results and Observations
Running the above script will insert various data types into the data_types collection and then retrieve them. The output will show the correct storage and retrieval of each data type.
Name: integer, Value: 123
Name: decimal, Value: 3.14
Name: string, Value: Hello, World!
Name: boolean, Value: True
Name: date, Value: 2025-05-21 23:26:24.971000
Name: timestamp, Value: 1747891584.972384
Name: array, Value: [1, 2, 3, 4, 5]
Name: object, Value: {'a': 1, 'b': 2}
Name: null, Value: None
Name: undefined, Value: None
I was not able to insert an instance of a custom class object. So those types of things would need to be serialized into a compatible format. When I tried inserting my class object, I got the error Invalid document...cannot encode object
.
Conclusion
In this article, we explored the various data types that can be stored in a MongoDB database using Python. We created a simple Python script that inserts different data types into a MongoDB collection and then retrieves them to verify their correctness. The results show that MongoDB supports a wide range of data types, including integers, floats, strings, booleans, dates, timestamps, arrays, objects, and null values. By understanding the data types supported by MongoDB, developers can design and implement efficient and effective data storage solutions for their applications.